Understanding JWT: A Comprehensive Guide to JSON Web Tokens
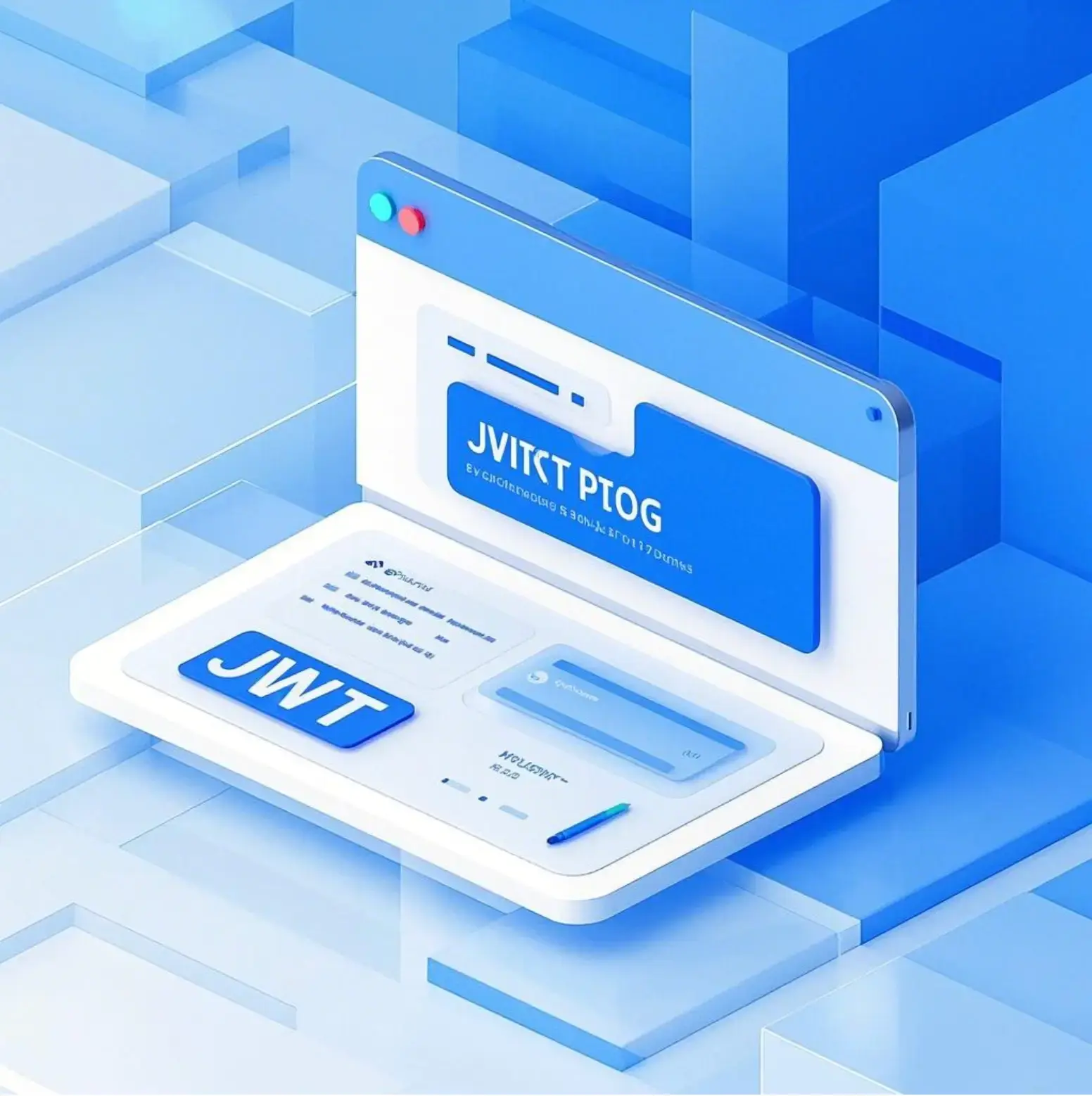
JSON Web Token (JWT) has become an essential technology in modern web development, particularly for authentication and information exchange. In this comprehensive guide, we’ll explore what JWTs are, how they work, and when to use them.
What is JWT?
JWT (JSON Web Token) is an open standard (RFC 7519) that defines a compact and self-contained way for securely transmitting information between parties as a JSON object. This information can be verified and trusted because it is digitally signed.
Structure of a JWT
A JWT consists of three parts, separated by dots (.):
- Header - Contains metadata about the token (type and signing algorithm)
- Payload - Contains the claims (token data)
- Signature - Verifies the token hasn’t been altered
Example of a JWT:
eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.
eyJzdWIiOiIxMjM0NTY3ODkwIiwibmFtZSI6IkpvaG4gRG9lIiwiaWF0IjoxNTE2MjM5MDIyfQ.
SflKxwRJSMeKKF2QT4fwpMeJf36POk6yJV_adQssw5c
How JWT Works
- The user logs in with their credentials
- The server validates the credentials and creates a JWT
- The server sends the JWT back to the client
- The client stores the JWT (usually in local storage)
- The client sends the JWT with subsequent requests
- The server validates the JWT signature and processes the request
Benefits of Using JWT
- Stateless Authentication: Servers don’t need to store session information
- Cross-domain/CORS: Tokens can be used across multiple domains
- Performance: No database lookups needed for authentication
- Decentralized: Perfect for microservices architectures
- Mobile Ready: Works well with native mobile apps
Security Considerations
While JWTs are secure by design, there are several best practices to follow:
- Use HTTPS: Always transmit JWTs over HTTPS
- Choose Strong Secret Keys: Use long, random strings for signing
- Set Appropriate Expiration: Use short-lived tokens
- Validate All Claims: Always verify token claims on the server
- Store Tokens Securely: Never store sensitive JWTs in local storage
Common Use Cases
- Authentication: Most common use case for JWTs
- Information Exchange: Secure way to transmit data between parties
- Authorization: Control access to protected resources
- Single Sign-On: Enable cross-domain authentication
Implementation Example
Here’s a simple example using Node.js and the jsonwebtoken
library:
const jwt = require('jsonwebtoken');
// Creating a token
const token = jwt.sign(
{ userId: '123', role: 'admin' },
'your-secret-key',
{ expiresIn: '1h' }
);
// Verifying a token
try {
const decoded = jwt.verify(token, 'your-secret-key');
console.log(decoded);
} catch(err) {
console.error('Token verification failed:', err.message);
}
Best Practices
- Keep Tokens Small: Include only necessary claims
- Use Short Expiration Times: Refresh tokens if needed
- Implement Token Revocation: Have a strategy for invalidating tokens
- Secure Token Storage: Use appropriate storage mechanisms
- Monitor Token Usage: Implement logging and monitoring
Common Pitfalls to Avoid
- Storing sensitive data in JWT payload
- Using weak secret keys
- Not validating tokens properly
- Storing tokens insecurely
- Using tokens for long-term sessions
Conclusion
JWT is a powerful tool for modern web applications, offering a secure and efficient way to handle authentication and information exchange. By following best practices and understanding its limitations, you can effectively implement JWT in your applications while maintaining security and performance.
Remember that while JWTs are powerful, they’re not suitable for every use case. Consider your specific requirements and security needs when deciding whether to use JWTs in your application.
Additional Resources
- JWT.io - Official JWT website and debugger
- RFC 7519 - JWT specification
- OWASP JWT Security - Security testing guide